Django란?
파이썬으로 만들어진 무료 오픈소스 웹 애플리케이션 프레임워크(web application framework)입니다.
Django 구조
이미지 출처: https://velog.io/@monsterkos/TIL-2020.06.05
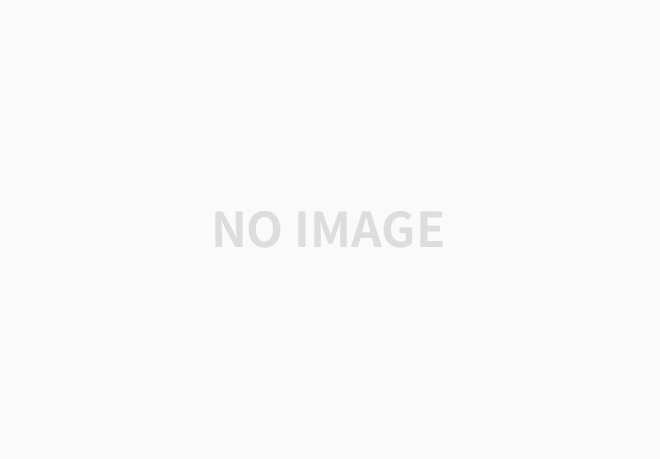
MTV 디자인 패턴
Model: database 스키마를 정의하고 CRUD를 가능하게 한다
Templates: 사용자 UI (html)
View: 서버, 모든 로직을 처리하는 controller, 사용자의 요청은 url에 따라 서버에 있는 함수를 실행 시키고 model에 접근할 수도 templates에 접근할 수도 있다
Django 사용법
1. 프로젝트를 담을 폴더 생성
컴퓨터 적당한 디렉토리를 골라 폴더 하나를 만든다. |
2. 가상환경 생성
copy pythonpython -m venv myvenv # myvenv는 가상환경 이름이다 myvenv가 아니여도 된다
가상환경이란?
djanngo를 실행하기 위해 필요한 서비스 패키지들을 설치해야하는데 프로젝트마다 필요한 패키지의 종류와 버전이 다를 수 있다. 그렇기 때문에 각 프로젝트 마다 가상환경을 만들어 필요한 패키지와 버전을 설치 할 공간이 필요한데 그것이 가상환경이다 |
가상환경을 사용하는 이유?
두가지 django 프로젝트를 개발한다고 가정하자. A는 파이썬 2.7버전 B는 파이썬 3.7버전을 사용한다. 그렇다면 a프로젝트를 개발 할때 개발자 컴퓨터에는 파이썬 2.7버전을 설치해야 한다. 그리고 같은 컴퓨터에서 B프로젝트를 개발하려고 한다면 파이썬 3.7버전이 필요하기 때문에 기존의 2.7버전을 삭제하고 3.7버전을 설치해야하는 번거로움이 생길 수 있다. 프로젝트가 커질 수록 필요한 패키지들이 많아지는데 수십가지의 패키지의 버전을 일일이 확인하고 맞추는것이 사실상 불가능하기 떄문에 가상환경을 사용한다. |
3. 가상환경 실행
copy pythonsource myvenv/Scripts/activate # . myvenv/Scripts/activate 와 동일
myvenv가 있는 디렉토리에서 가상환경을 실행하면 그 이후에 설치되는 모든 패키지는 해당 가상환경에 포함되게 된다.
4. django 설치
copy pythonpip3 install django
5. 프로젝트 생성
copy pythondjango-admin startproject 프로젝트이름
django를 설치 했다면 이제 프로젝트를 만들어야한다. 위의 명령어를 실행하고 나면 django 개발환경이 자동으로 구축된다
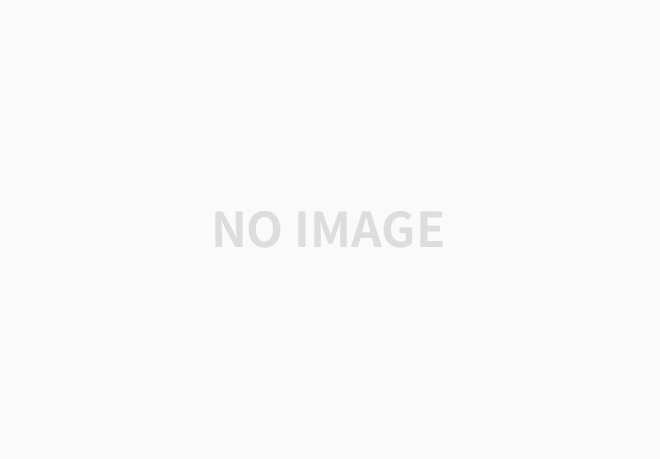
6. app 생성
copy pythonpython manage.py startapp 앱이름
app은 하나의 전체 프로젝트에서 부분적안 역할 맡고 있는 한 기능이라고 생각하면 된다.
예를 들어 블로그를 만든다고 해보자 블로그는 블로그의 소식과 광고를 보여주는 메인페이지, 사람들이 글을 쓰고 볼수 있는 게시판 2가지로 구성한다고 해보자. 그렇다면 메인페이지 기능을 할 mainsite app 한개, 게시판 board app 한개를 만들면 된다 |
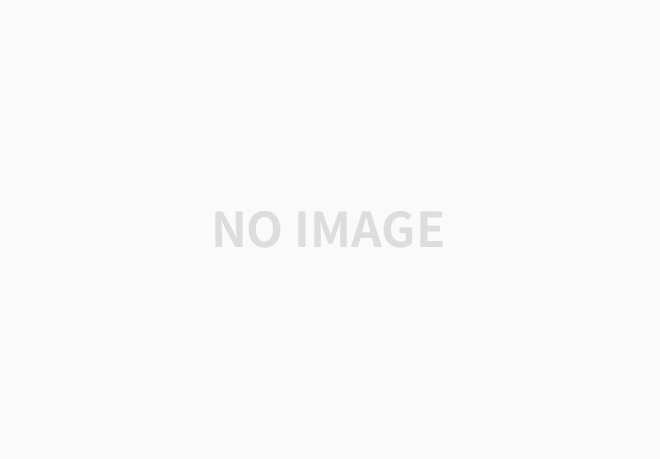
위의 사진은 media를 재외하고 총 6개의 app이 있다. 여기서 보면 프로젝트 이름과 똑같은 이름을 가진 app이 하나 있는데 이것은 프로젝트를 만들면 자동으로 만들어주는 app이다.
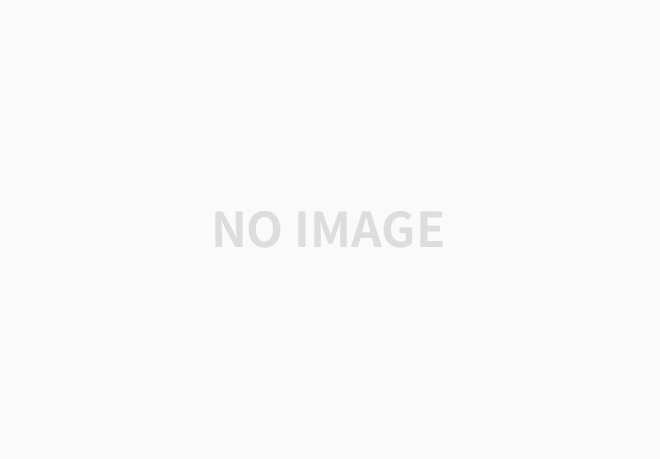
settings.py
프로젝트의 설정 정보를 담고 있는 파일 |
urls.py
copy pythonfrom django.contrib import admin
from django.urls import path, include
from django.conf.urls.static import static# 2 미디어 static 사용
from django.conf import settings
urlpatterns = [
path('admin/', admin.site.urls),
path('festival/', include('festival.urls')), # festival app의urls.py
path('album/', include('album.urls')), # album app의urls.py
path('board/', include('board.urls')), # board app의urls.py
path('', include('likelion.urls')), # likelion app의urls.py
]
if settings.DEBUG:
urlpatterns += static(settings.MEDIA_URL, document_root=settings.MEDIA_ROOT)
url은 사용자가 특정 페이지에 접근하기 위해 필요한 주소이다. path() 안에 특정 url로 시작하는 요청이 들어오면 특정 app의 urls.py로 이동하겠다는 의미이다. 사용자가 처음으로 사이트를 접속할때 바로 이곳, 프로젝트를 만들면 자동으로 만들어주는 app에 있는 urls.py로 요청이 들어오게 된다. |
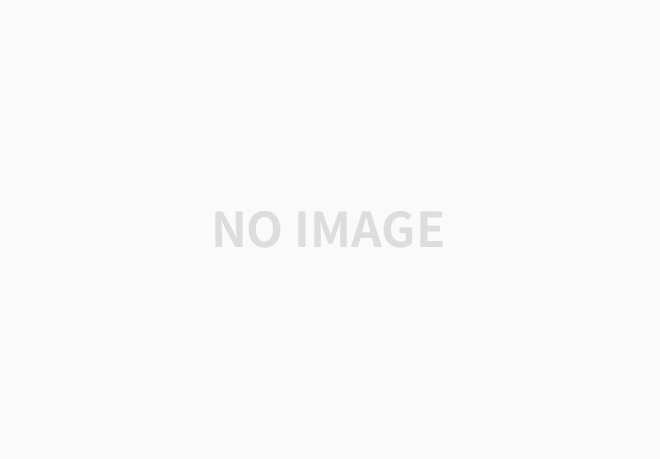
copy pythonfrom django.urls import path
from django.conf import settings
from django.conf.urls.static import static
from . import views
urlpatterns = [
path('', views.board, name='board'), # board/ 로 요청이 왔을때 views.board를 실행
path('<int:pk>/', views.board_view, name='board_view'), #board/숫자 로 요청이 왔을 때 views.board_view 실행
path('new/', views.board_create, name='board_create'), # board/new로 요청이 왔을때views.board_create 실행
path('update/<int:pk>/', views.board_update, name='board_update'), #board/update/숫자 로 요청이 왔을 때 ...
path('delete/<int:pk>/', views.board_delete, name='board_delete'),
path('search/', views.board_search, name='board_search'),
path('check/', views.check_password, name='check_password'),
]
if settings.DEBUG:
urlpatterns += static(settings.MEDIA_URL, document_root=settings.MEDIA_ROOT)
'board/new' 라는 url로 접속했다고 하고 board 앱을 보자. 여기에도 urls.py가 있는것을 볼 수 있다. 여기서는 'board/.../.../...' 로 시작하는 url을 관리하는 곳이다. 사용법은 코드의 주석을 참고하길 바란다. |
7. model 사용법
copy pythonfrom django.db import models
# Create your models here.
class Board(models.Model):
title = models.CharField(max_length=125)
post_type = models.CharField(max_length=125, default="question")
content = models.TextField()
author = models.CharField(max_length=125, null=True)
password = models.CharField(max_length=15)
lookup = models.IntegerField(default=0)
created_at = models.DateTimeField(auto_now_add=True)
updated_at = models.DateTimeField(auto_now=True)
board app의 models.py로 가보자 class 테이블명 (models.Model)으로 테이블을 정의하고 안에 내용은 칼럼들이다. CharField는 char형 TextField는 길이가 긴 char형 IntegerField 는 number형이다 괄호안에는 제약조건들을 명시할 수 있다. |
8. views 사용법
copy pythonfrom django.shortcuts import render, redirect
from django.utils import translation, timezone
from django.http import HttpResponseRedirect
from django.urls import reverse
from .models import Board
from django.core.paginator import Paginator, EmptyPage, PageNotAnInteger
# Create your views here.
# show board list
def board(request):
boards = Board.objects.all()
paginator = Paginator(boards, 5) # Show 25 contacts per page
page = request.GET.get('page')
try:
contacts = paginator.page(page)
except PageNotAnInteger:
# If page is not an integer, deliver first page.
contacts = paginator.page(1)
except EmptyPage:
# If page is out of range (e.g. 9999), deliver last page of results.
contacts = paginator.page(paginator.num_pages)
context = {'contacts': contacts, 'site': "board"}
return render(request, 'board/board.html', context)
# show board
def board_view(request, pk):
board = Board.objects.get(pk=pk)
board.lookup += 1
board.save()
context = {'board':board, 'site': "board"}
return render(request, 'board/board_view.html', context)
# create board
def board_create(request):
if request.method == 'POST':
author = request.POST.get('author')
password = request.POST.get('password')
post_type = request.POST.get('post_type')
title = request.POST.get('title')
content = request.POST.get('content')
board = Board(
author = author,
password = password,
post_type = post_type,
title = title,
content = content
)
board.save()
context = {'board': board, 'site': "board"}
return render(request,'board/board_view.html', context)
return render(request, 'board/new_board.html')
# search board
def board_search(request):
boards = Board.objects.all()
post_type = request.GET.get('type')
search = request.GET.get('search')
page = request.GET.get('page')
error = False
if post_type != None:
request.session['post_type'] = post_type
request.session['search'] = search
try:
if request.session['post_type'] == "all":
boards = boards.filter(title__icontains = request.session['search'])
elif request.session['search'] == "":
boards = boards.filter(post_type = request.session['post_type'])
else:
boards = boards.filter(post_type = request.session['post_type'], title__icontains = request.session['search'])
paginator = Paginator(boards, 5) # Show 25 contacts per page
contacts = paginator.page(page)
except PageNotAnInteger:
# If page is not an integer, deliver first page.
contacts = paginator.page(1)
except EmptyPage:
# If page is out of range (e.g. 9999), deliver last page of results.
contacts = paginator.page(paginator.num_pages)
if boards.exists():
message = "게시글을 찾았습니다."
else:
message = "해당 게시글이 없습니다."
error = True
context = {'boards':boards,'contacts': contacts, 'message':message, 'error':error, 'site': "board"}
return render(request, 'board/board.html', context)
# update board
def board_update(request):
board = Board.objects.get(pk = request.POST.get('pk'))
board.post_type = request.POST.get('post_type')
board.title = request.POST.get('title')
board.content = request.POST.get('content')
board.updated_at = timezone.now()
board.save()
context = {'board':board, 'site': "board"}
return render(request,'board/board_view.html', context)
# delete board
def board_delete(request, pk):
board = Board.objects.get(pk = pk)
board.delete()
return redirect(reverse('board'))
board app의 views.py로 가보자 기본적인 crud 를 구현해 보았다. def 함수명(request, ...): 는 위에 urls.py에서 특정 url로 요청이 들어오면 views에 있는 함수를 실행한다고 했는데 그 함수들을 만들어 놓은 것이다. |
'Django' 카테고리의 다른 글
Django dbsqlite3 오류 (0) | 2019.09.23 |
---|---|
django email 라이브러리 (0) | 2019.09.23 |
django excel 불러오기 및 생성 하기 (0) | 2019.09.23 |
Django 명령어 모음 (0) | 2019.09.23 |